Modify a Message
Feature Description
This feature enables any participant in a conversation to modify a successfully sent message in the conversation. The message will be synced to all the participants in the conversation once modified successfully.
Note:
This feature is supported only by the Enhanced edition on v6.2 or later.
Effect
You can use this API to change the
cloudCustomData
of a message, implementing features such as message reply and message quote as shown below: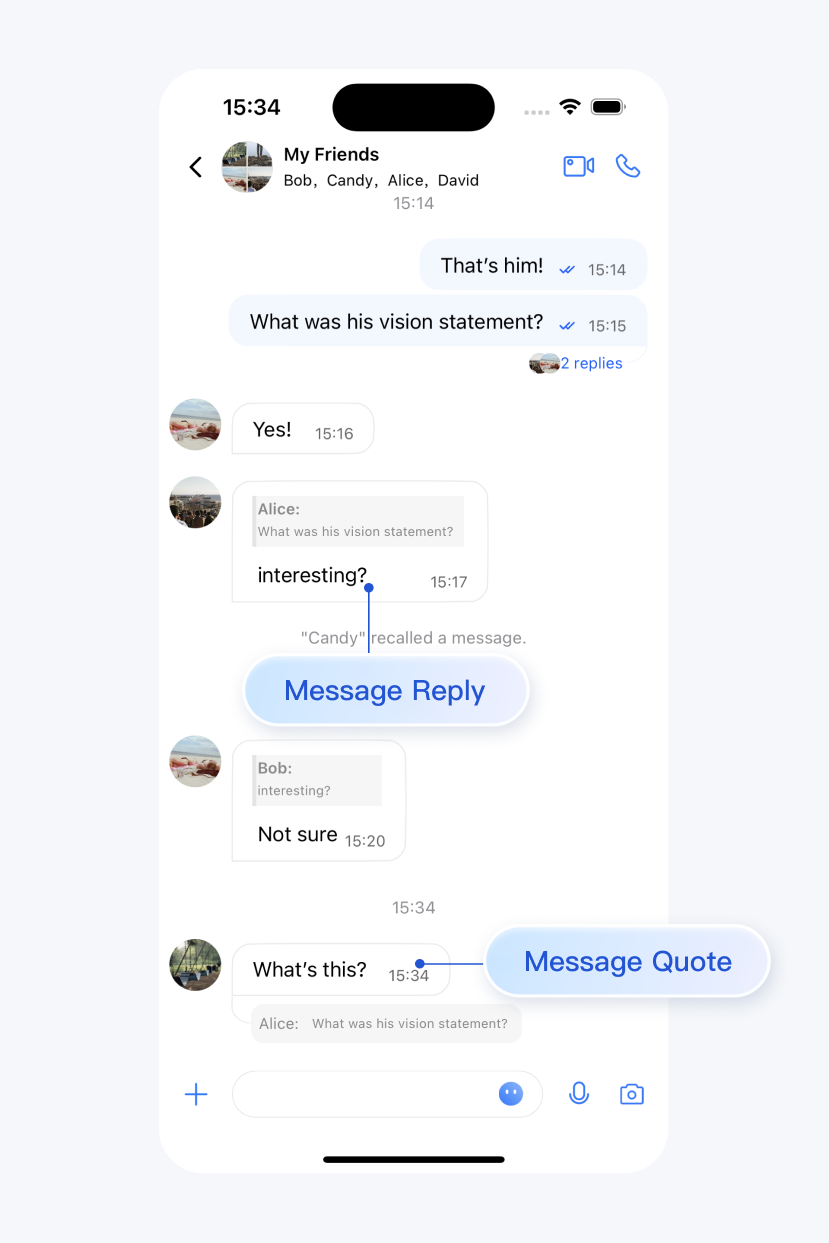
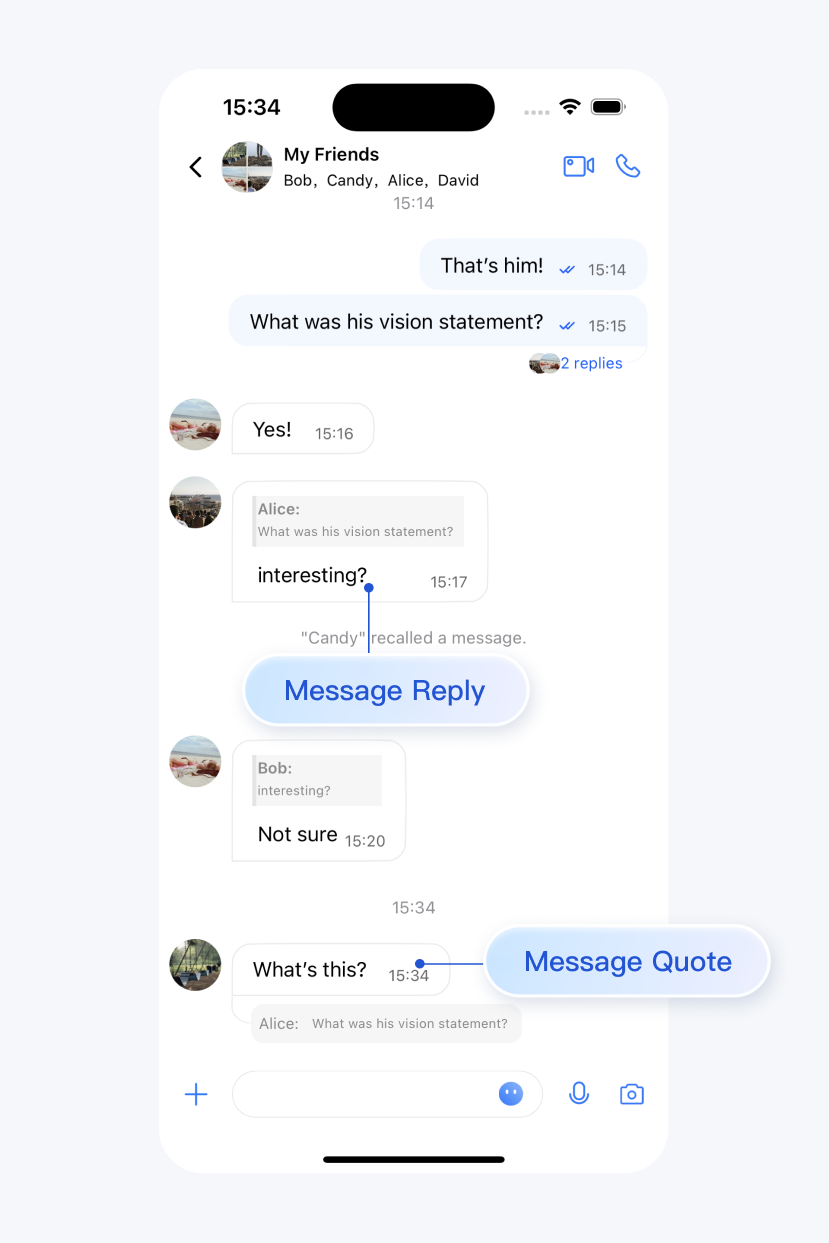
API Description
Modifying a Message
A conversation participant can call
modifyMessage
(Java / Swift / Objective-C / C++) to modify a sent message in the conversation.
The SDK allows any conversation participant to modify a message in the conversation. You can add more restrictions at the business layer, for example, only allowing the message sender to modify the message.Currently, the following information of a message can be modified:
cloudCustomData
(Java / Swift / Objective-C / C++) V2TIMTextElem
(Java / Swift / Objective-C / C++) V2TIMCustomElem
(Java / Swift / Objective-C / C++)V2TIMLocationElem
(Java / Swift / Objective-C/ C++)V2TIMFaceElem
(Java / Swift / Objective-C / C++)Sample code:
// The original message object in the conversation is `originMessage`.// Modify the `cloudCustomData` information of the message objectoriginMessage.setCloudCustomData("modify_cloud_custom_data".getBytes());// If the message is a text message, modify the text message contentif (V2TIMMessage.V2TIM_ELEM_TYPE_TEXT == originMessage.getElemType()) {originMessage.getTextElem().setText("modify_text");}V2TIMManager.getMessageManager().modifyMessage(originMessage, new V2TIMCompleteCallback<V2TIMMessage>() {@Overridepublic void onComplete(int code, String desc, V2TIMMessage message) {// After the message is modified, `message` is the modified message object.}});
// The original message object in the conversation is `originMessage`.var originMessage: V2TIMMessage!// Modify the `cloudCustomData` information of the message objectoriginMessage.cloudCustomData = "modify_cloud_custom_data".data(using: .utf8)// If the message is a text message, modify the text message contentif originMessage.elemType == .V2TIM_ELEM_TYPE_TEXT {originMessage.textElem?.text = "modify_text"}// modifyMessageV2TIMManager.shared.modifyMessage(msg: originMessage) { code, desc, msg in// After the message is modified, `msg` is the modified message object.}
// Original message object in the conversationV2TIMMessage *originMessage;// Modify the `cloudCustomData` information of the message objectoriginMessage.cloudCustomData = [@"modify_cloud_custom_data" dataUsingEncoding:NSUTF8StringEncoding];// If the message is a text message, modify the text message contentif (V2TIM_ELEM_TYPE_TEXT == originMessage.elemType) {originMessage.textElem.text = @"modify_text";}[[V2TIMManager sharedInstance] modifyMessage:originMessage completion:^(int code, NSString *desc, V2TIMMessage *msg) {// After the message is modified, `msg` is the modified message object.}];
template <class T>class CompleteCallback final : public V2TIMCompleteCallback<T> {public:using InternalCompleteCallback =std::function<void(int, const V2TIMString&, const T&)>;CompleteCallback() = default;~CompleteCallback() override = default;void SetCallback(InternalCompleteCallback complete_callback) { complete_callback_ = std::move(complete_callback); }void OnComplete(int error_code, const V2TIMString& error_message, const T& value) override {if (complete_callback_) {complete_callback_(error_code, error_message, value);}}private:InternalCompleteCallback complete_callback_;};// V2TIMMessage originMessage;std::string str = u8"modify_cloud_custom_data";// Modify the `cloudCustomData` information of the message objectoriginMessage.cloudCustomData = {reinterpret_cast<const uint8_t*>(str.data()), str.size()};if (originMessage.elemList.Size() == 1) {V2TIMElem* elem = originMessage.elemList[0];if (elem->elemType == V2TIMElemType::V2TIM_ELEM_TYPE_TEXT) {// If the message is a text message, modify the text message contentauto textElem = static_cast<V2TIMTextElem*>(elem);textElem->text = "modify_text";}}auto callback = new CompleteCallback<V2TIMMessage>{};callback->SetCallback([=](int error_code, const V2TIMString& error_message, const V2TIMMessage& message) {// After the message is modified, `message` is the modified message object.delete callback;});V2TIMManager::GetInstance()->GetMessageManager()->ModifyMessage(originMessage, callback);
Message Modified Notification
Conversation participants call
addAdvancedMsgListener
(Java / Swift / Objective-C / C++) to add the advanced message listener.After a message in the conversation is modified, all the participants will receive the
onRecvMessageModified
callback (Java / Swift / Objective-C / C++), which contains the modified message object.Sample code:
V2TIMAdvancedMsgListener advancedMsgListener = new V2TIMAdvancedMsgListener() {// Notification of the message content modification@Overridepublic void onRecvMessageModified(V2TIMMessage msg) {// `msg` is the modified message object.}};// Add a message listenerV2TIMManager.getMessageManager().addAdvancedMsgListener(advancedMsgListener);
// Add a message listenerV2TIMManager.sharedInstance().addAdvancedMsgListener(self)/// Notification of the message content modificationfunc onRecvMessageModified(_ msg: V2TIMMessage) {// `msg` is the modified message object.}
// Add a message listener[[V2TIMManager sharedInstance] addAdvancedMsgListener:self];/// Notification of the message content modification- (void)onRecvMessageModified:(V2TIMMessage *)msg {// `msg` is the modified message object.}
class AdvancedMsgListener final : public V2TIMAdvancedMsgListener {public:// Notification of the message content modificationvoid OnRecvNewMessage(const V2TIMMessage& message) override {// `message` is the modified message object.}// Other members ...};// Note that `advancedMsgListener` should not be released before the IM SDK is uninitialized,// otherwise the message callback cannot be called.AdvancedMsgListener advancedMsgListener;V2TIMManager::GetInstance()->GetMessageManager()->AddAdvancedMsgListener(&advancedMsgListener);