Live Chat
Description of the Feature
The interactive barrage feature is a crucial real-time communication tool, supporting various interactive methods. Users can input emojis in the barrage to enhance the entertainment value of messages, making the interactive experience more enjoyable and vivid. Through this feature, the audience can engage in richer communication with anchors and other viewers during live streaming, boosting the overall sense of participation and fun. The TUILiveKit has already implemented the interactive barrage feature through Chat.
Use Instructions
Display Barrage | Send Barrage |
![]() | ![]() |
Note:
Supports switching between System Keyboard and Emoji Keyboard.
Feature Customization
Custom barrage message style from Definition
There are two styles of Danmaku messages: Ordinary Danmaku Message Style and Gift Sending Echo Message Style.
If you need to define the Ordinary Danmaku Message Style, please refer to the following path for changes:
// File Location: iOS/TUILiveKit/Source/Common/UIComponent/Barrage/View/Cell/TUIBarrageCell.swiftclass TUIBarrageDefaultCell: UIView {// Ordinary Danmaku Message Style...func constructViewHierarchy() {// View Hierarchy Construction}func activateConstraints() {// View Layout}}
If you need to modify the Gift Sending Echo Message Style, please refer to the following path for changes:
// File Location: iOS/TUILiveKit/Source/Common/UIComponent/Gift/View/CustomBarrageCell.swiftclass CustomBarrageCell {static func getCustomCell(barrage: TUIBarrage) -> UIView {// Returns the UI style for gift echo messages}}
TUIBarrage
Definition is as follows:// File Location:// iOS/TUILiveKit/Source/Common/UIComponent/Barrage/Model/TUIBarrage.swift// iOS/TUILiveKit/Source/Common/UIComponent/Barrage/Model/TUIBarrageUser.swiftclass TUIBarrage: Codable{var user: TUIBarrageUservar content: Stringvar extInfo: [String: AnyCodable]}class TUIBarrageUser: Codable {var userId: Stringvar userName: Stringvar avatarUrl: Stringvar level: String}
Insert custom messages
The barrage display component
TUIBarrageDisplayView
provides the insertBarrages
interface method for (batch) inserting custom Definition messages. Typically, custom Definition messages combined with custom styles achieve unique display effects.// File Location:// iOS/TUILiveKit/Source/View/LiveRoom/View/Anchor/LivingView.swift// iOS/TUILiveKit/Source/View/LiveRoom/View/Anchor/AudienceLivingView.swift// Example: Inserting a gift message in the barrage arealet barrage = TUIBarrage()barrage.content = "gift"barrage.user.userId = sender.userIdbarrage.user.userName = sender.userNamebarrage.user.avatarUrl = sender.avatarUrlbarrage.user.level = sender.levelbarrage.extInfo["TYPE"] = AnyCodable("GIFTMESSAGE")barrage.extInfo["gift_name"] = AnyCodable(gift.giftName)barrage.extInfo["gift_count"] = AnyCodable(giftCount)barrage.extInfo["gift_icon_url"] = AnyCodable(gift.imageUrl)barrage.extInfo["gift_receiver_username"] = AnyCodable(receiver.userName)barrageDisplayView.insertBarrages([barrage])
Note:
TUIBarrage
's extInfo
is a Map
, used to store custom data.Key code
Quick Integration
The barrage component mainly provides 2 APIs:
TUIBarrageButton
: Clicking it brings up the input interface.TUIBarrageDisplayView
: Used to display barrage messages.In scenarios where you need to send barrages, create
TUIBarrageButton
, click to bring up the input interface:let barrageButton = TUIBarrageButton(roomId: roomId, ownerId: ownerId) addSubView(barrageButton)
In scenarios where you need to display barrages, use
TUIBarrageDisplayView
to display barrage messages:let barrageDisplayView = TUIBarrageDisplayView(roomId: roomId, ownerId: ownerId) addSubView(barrageDisplayView)
Sending and Receiving Barrage Messages
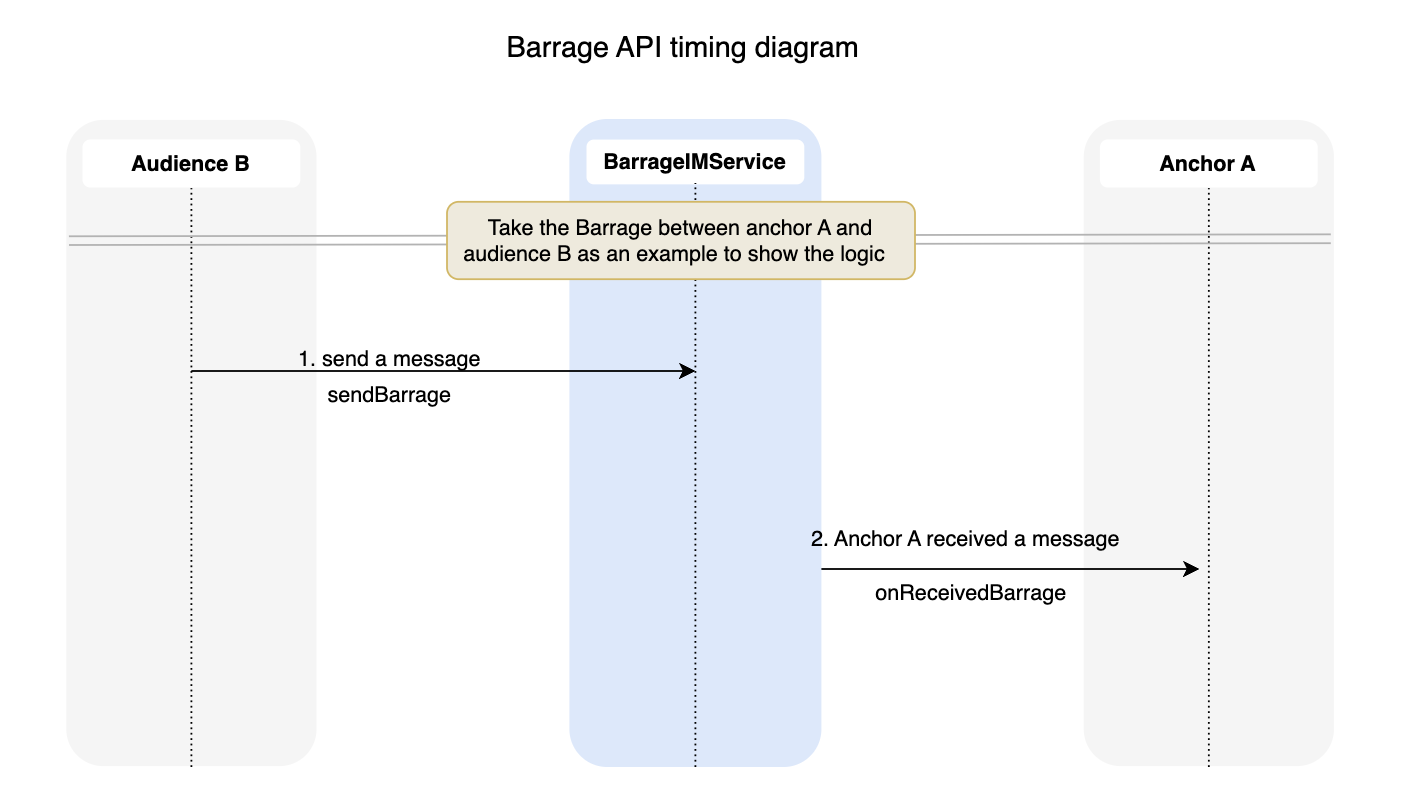
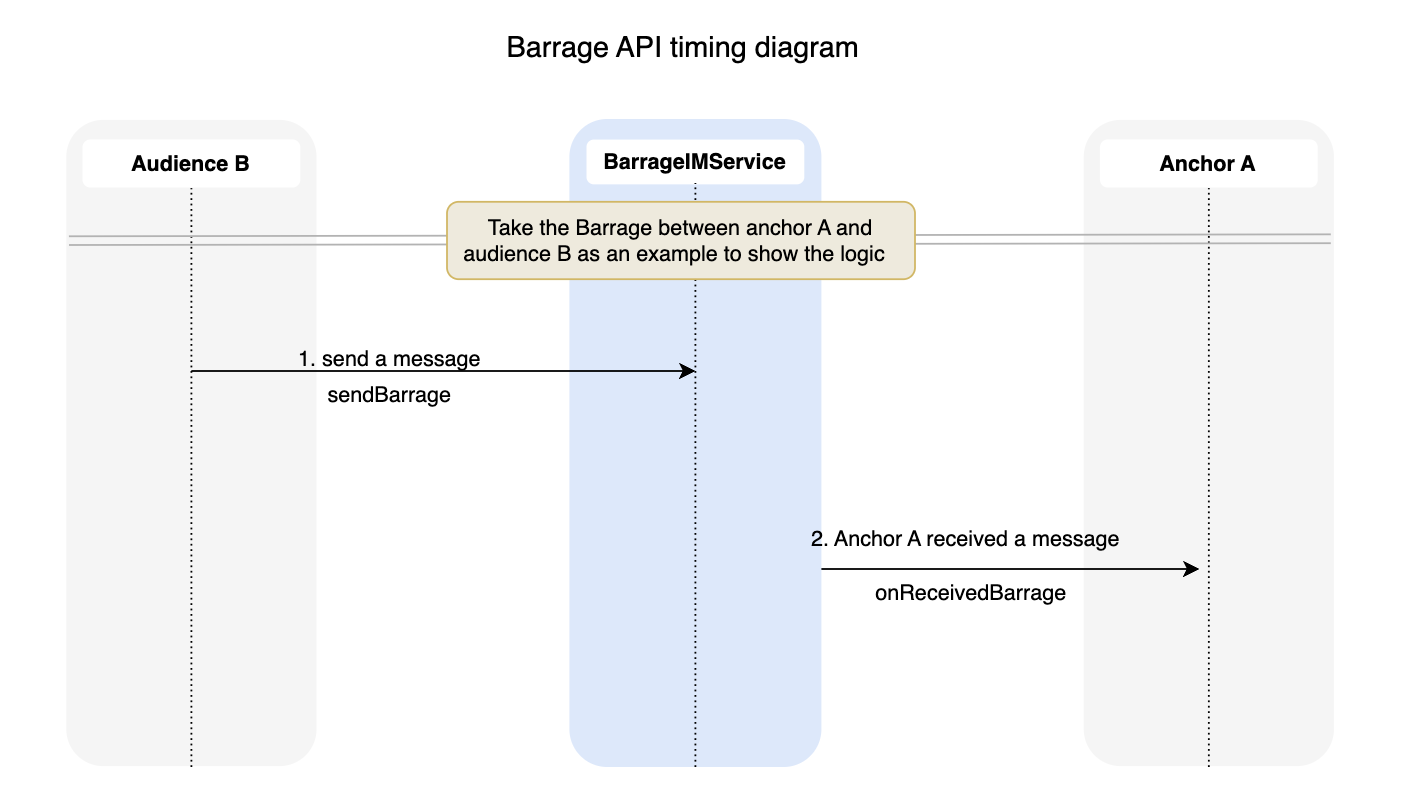