Flutter
Feature Description
A community is a large group of people brought together by common topics, and multiple topics can be created under the same community based on different interests.
Community groups are used to manage group members. All topics under the same community group are shared among members, who can send and receive messages within each topic independently.
See use cases of community groups here.
Community and topic management APIs are in the
TencentImSDKPlugin.v2TIMManager.getGroupManager()
core class.Topic message APIs are in the
TencentImSDKPlugin.v2TIMManager.getMessageManager()
core class.Note:
This feature is supported by Flutter SDK v4.0.0 or later. To use it, you need to purchase the Ultimate edition, go to the console, choose Feature Configuration > Group configuration > Group feature configuration > Community, and enable the community feature.
Community Group Management
Creating a community group
You need to perform two steps to create a community group that supports topics:
1. Create the
V2TIMGroupInfo
object (Details) and set groupType
to Community
and isSupportTopic
to true
/YES
.2. Call the
createGroup
(Details) API to create the community group.Sample code:
// Create a topic-enabled communitygroupManager.createGroup(groupType: "Community", groupName: "Community",isSupportTopic: true);
Getting the list of community groups joined
Sample code:
// Getting the list of community groups joinedV2TimValueCallback<List<V2TimGroupInfo>> groupList = await groupManager.getJoinedCommunityList();
Other management APIs
Other features can be used in the same way as an ordinary group feature and involve the following APIs:
Category | Feature | API |
Community group management | ||
| ||
| ||
| ||
| ||
Community group member management | ||
| ||
| ||
|
Topic Management
Multiple topics can be created under the same community group. All the topics are shared among group members, who can send and receive messages within each topic independently.
Note:
To use the feature, you need to go to the console, choose Feature Configuration > Group configuration > Group feature configuration > Community, enable the community feature and then enable the topic feature.
Creating a topic
You need to perform two steps to create a topic:
1. Create a
V2TIMTopicInfo
(Details) object.2. Call the
createTopicInCommunity
(Details) API to create a topic.Sample code:
// Create a topicgroupManager.createTopicInCommunity(groupID: "groupID", topicInfo: V2TimTopicInfo.fromJson({"topicName":"topic"}));
Deleting a topic
Sample code:
// Delete a topicgroupManager.deleteTopicFromCommunity(groupID: "",topicIDList:["topicID"]);
Modifying topic information
You need to perform two steps to modify the information of a topic:
1. Create a
V2TIMTopicInfo
(Details) object and modify fields as needed.2. Call the
setTopicInfo
(Details) API to modify topic information.Sample code:
// Modify topic informationgroupManager.setTopicInfo(topicInfo:V2TimTopicInfo.fromJson({"topicName":"topicName"}));
Getting the topic list
If
topicIDList
is an empty array, the list of all topics of the community group will be got.If
topicIDList
is the ID of specified topics, the list of the specified topics will be got.Sample code:
// Get the topic listgroupManager.getTopicInfoList(groupID: "",topicIDList: ['topicID']);
Topic groups
The community is a new powerful tool for entertainment collaboration and supports the community-group-topic hierarchy to isolate messages.
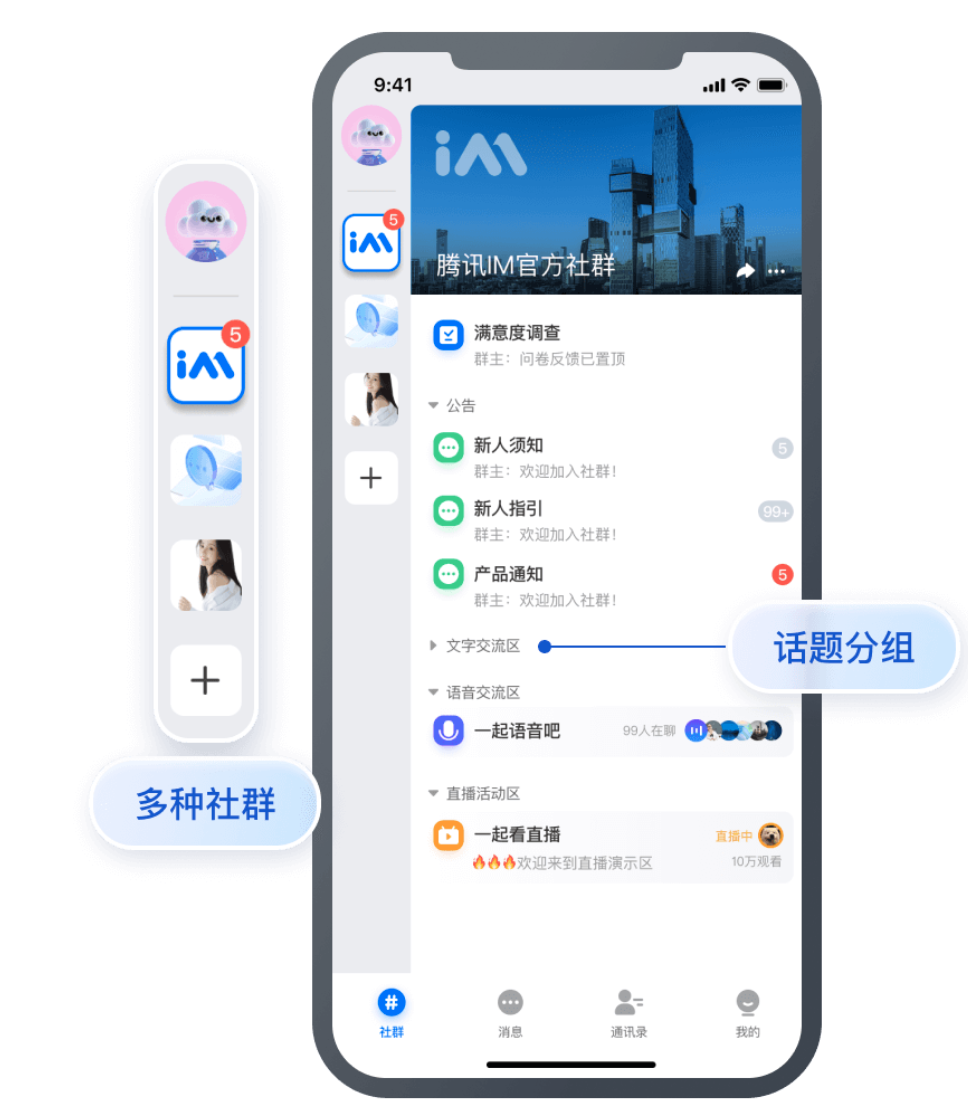
The
customInfo
of a community saves the topic group list of the community, while the customString
field of each topic stores the topic group.When a community is loaded, the
customInfo
field for the topic group list of the community (group) is used to display the group list. We recommend you store the field in the List<String>
format.To get the topics in each group, traverse the topic list and get the group of each topic through the
customString
of V2TimTopicInfo
.Note:
You can customize the
key
value of the customInfo
field for the topic group list of the community (group).
The following sample code names it topic_category
.Getting the list of groups in the community
Call the
getCommunityCategoryList(String groupID)
method. Sample code:getCommunityCategoryList(String groupID) async {final Map<String, String>? customInfo = await getCommunityCustomInfo(groupID);if(customInfo != null){final String? categoryListString = customInfo["topic_category"];if(categoryListString != null && categoryListString.isNotEmpty){return jsonDecode(categoryListString);}}}Future<Map<String, String>?> getCommunityCustomInfo(String groupID) async {V2TimValueCallback<List<V2TimGroupInfoResult>> res =await TencentImSDKPlugin.v2TIMManager.getGroupManager().getGroupsInfo(groupIDList: [groupID]);if(res.code != 0){final V2TimGroupInfoResult? groupInfo = res.data?[0];if(groupInfo != null){Map<String, String>? customInfo = groupInfo.groupInfo?.customInfo;return customInfo;}}return null;}
Configuring the group list for the community
You just need to modify the
customInfo
in groupInfo
. Here is a Map
, and the key
value is the name of the field for the topic group list you defined.The
getCommunityCustomInfo
method is implemented in the above section. Sample code:setCommunityCategoryList(String groupID, String groupType, List<String> newCategoryList) async {final Map<String, String>? customInfo = await getCommunityCustomInfo(groupID);customInfo?["topic_category"] = jsonEncode(newCategoryList);TencentImSDKPlugin.v2TIMManager.getGroupManager().setGroupInfo(info: V2TimGroupInfo(customInfo: customInfo,groupID: groupID,groupType: groupType,// ...Other profiles));}
Adding a topic to a group
It is recommended that you pass in JSON strings into
V2TimTopicInfo customString
.
For example, the recommended format for categoryName
in the code below is {"category":"Group 1"}
.Sample code:
addCategoryForTopic(String groupID, String categoryName) {TencentImSDKPlugin.v2TIMManager.getGroupManager().setTopicInfo(topicInfo: V2TimTopicInfo(customString: categoryName),groupID: groupID, // Group ID of the topic);}
Getting the topic group
Listening for topic callbacks
In
V2TIMGroupListener
(Details), topic related callback methods onTopicCreated
, onTopicDeleted
, and onTopicInfoChanged
are added for topic event listening. Sample code:
V2TIMGroupListener v2TIMGroupListener = new V2TIMGroupListener() {onTopicCreated(String groupID, String topicID) {// Listen for topic creation notifications}onTopicDeleted(String groupID, List<String> topicIDList) {// Listen for topic deletion notifications}onTopicInfoChanged(String groupID, V2TIMTopicInfo topicInfo) {// Listen for topic information update notifications}};V2TIMManager.getInstance().addGroupListener(v2TIMGroupListener);
Topic Messages
Topic messages can be used in the same way as ordinary messages and involve the following APIs:
Feature | API | Description |
Sends a message | Set `groupID` to the topic ID. | |
Receives a message | Set `groupID` in the message to the topic ID. | |
Marks a message as read | Set `groupID` to the topic ID. | |
Gets historical messages | Set `groupID` to the topic ID. | |
Recalls a message | Set `groupID` to the topic ID. |