Integrating TUIVoiceRoom (Android)
Overview
TUIVoiceRoom
is an open-source audio/video UI component. After integrating it into your project, you can make your application support the group audio chat scenario simply by writing a few lines of code. It also supports the iOS platform. Its basic features are as shown below:Note
![]() |
Integration
Step 1. Download and import the TUIVoiceRoom
component
Go to GitHub, clone or download the code, copy the
Android/Source
directory to your project, and complete the following import operations:Add the code below in
setting.gradle
:include ':Source'
Add dependencies on
Source
to the build.gradle
file in app
:api project(':Source')
Add the TRTC SDK (
liteavSdk
) and Chat SDK (imSdk
) dependencies in build.gradle
in the root directory:ext {liteavSdk = "com.tencent.liteav:LiteAVSDK_TRTC:latest.release"imSdk = "com.tencent.imsdk:imsdk-plus:latest.release"}
Step 2. Configure permission requests and obfuscation rules
Configure permission requests for your app in
AndroidManifest.xml
. The SDKs need the following permissions (on Android 6.0 and later, the mic access must be requested at runtime):<uses-permission android:name="android.permission.INTERNET" /><uses-permission android:name="android.permission.ACCESS_NETWORK_STATE" /><uses-permission android:name="android.permission.RECORD_AUDIO" />
In the
proguard-rules.pro
file, add the SDK classes to the "do not obfuscate" list.-keep class com.tencent.** { *;}
Step 3. Initialize and log in to the component
// 1. InitializeTRTCVoiceRoom mTRTCVoiceRoom = TRTCVoiceRoom.sharedInstance(this);mTRTCVoiceRoom.setDelegate(new TRTCVoiceRoomDelegate() {);// 2. Log inmTRTCVoiceRoom.login(SDKAppID, userId, userSig, new TRTCVoiceRoomCallback.ActionCallback() {@Overridepublic void onCallback(int code, String msg) {if (code == 0) {// Logged in}}});
Parameter description:
SDKAppID: The TRTC application ID. If you haven't activated TRTC, log in to the TRTC console, create a TRTC application, click Application Info, and select the Quick Start tab to view its 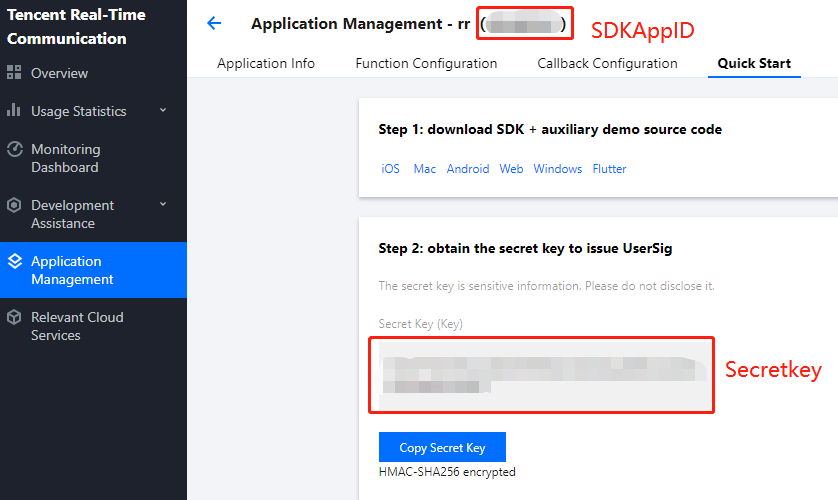
SDKAppID
.
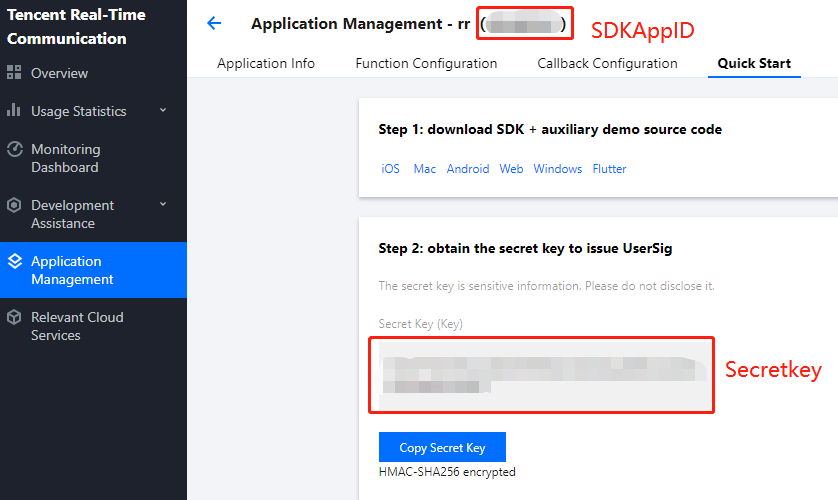
Secretkey: The TRTC application key. Each secret key corresponds to a
SDKAppID
. You can view your application’s secret key on the Application Management page of the TRTC console.userId: The ID of the current user, which is a string that can contain only letters (a-z and A-Z), digits (0-9), hyphens (-), and underscores (_). We recommend that you keep it consistent with your user account system.
userSig: The security protection signature calculated based on
SDKAppID
, userId
, and Secretkey
. You can click here to directly generate a debugging userSig
online. For more information, see UserSig.Step 4. Implement the audio chat room
1. The room owner creates an audio chat room through TRTCVoiceRoom#createRoom.
// 1. The room owner calls an API to create a roomint roomId = 12345; // Room IDfinal TRTCVoiceRoomDef.RoomParam roomParam = new TRTCVoiceRoomDef.RoomParam();roomParam.roomName = "Room name";roomParam.needRequest = false; // Whether the room owner’s permission is required for listeners to speakroomParam.seatCount = 7; // Number of room seats. In this example, the number is 7. 6 seats are available after you take one.roomParam.coverUrl = "URL of room cover image";mTRTCVoiceRoom.createRoom(roomId, roomParam, new TRTCVoiceRoomCallback.ActionCallback() {@Overridepublic void onCallback(int code, String msg) {if (code == 0) {// Room created successfully}}});
2. A listener enters the audio chat room through TRTCVoiceRoom#enterRoom.
// 1. A listener calls an API to enter the roommTRTCVoiceRoom.enterRoom(roomId, new TRTCVoiceRoomCallback.ActionCallback() {@Overridepublic void onCallback(int code, String msg) {if (code == 0) {// Entered room successfully}}});
3. A listener mics on through TRTCVoiceRoom#enterSeat.
// 1. A listener calls an API to mic onint seatIndex = 2; // Seat indexmTRTCVoiceRoom.enterSeat(seatIndex, new TRTCVoiceRoomCallback.ActionCallback() {@Overridepublic void onCallback(int code, String msg) {if (code == 0) {// Operation succeeded}}});// 2. The `onSeatListChange` callback is received, and the seat list is refreshed@Overridepublic void onSeatListChange(final List<TRTCVoiceRoomDef.SeatInfo> seatInfoList) {}
4. The room owner makes a listener a speaker through TRTCVoiceRoom#pickSeat.
// 1. The room owner makes a listener a speakerint seatIndex = 2; // Seat indexString userId = "123"; // The ID of the user who will become a speakermTRTCVoiceRoom.pickSeat(1, userId, new TRTCVoiceRoomCallback.ActionCallback() {@Overridepublic void onCallback(int code, String msg) {if (code == 0) {// Operation succeeded}}});// 2. The `onSeatListChange` callback is received, and the seat list is refreshed@Overridepublic void onSeatListChange(final List<TRTCVoiceRoomDef.SeatInfo> seatInfoList) {}
5. A listener requests to speak through TRTCVoiceRoom#sendInvitation.
// Listener// 1. A listener calls an API to request to speakString seatIndex = "1"; // Seat indexString userId = "123"; // User IDString inviteId = mTRTCVoiceRoom.sendInvitation("takeSeat", userId, seatIndex, null);// 2. Place the user in the seat after the invitation is accepted@Overridepublic void onInviteeAccepted(String id, String invitee) {if(id.equals(inviteId)) {mTRTCVoiceRoom.enterSeat(index, null);}}// Room owner// 1. The room owner receives the request@Overridepublic void onReceiveNewInvitation(final String id, String inviter, String cmd, final String content) {if (cmd.equals("takeSeat")) {// 2. The room owner accepts the requestmTRTCVoiceRoom.acceptInvitation(id, null);}}
6. The room owner invites a listener to speak through TRTCVoiceRoom#sendInvitation.
// Room owner// 1. The room owner calls an API to invite a listener to speakString seatIndex = "1"; // Seat indexString userId = "123"; // User IDString inviteId = mTRTCVoiceRoom.sendInvitation("pickSeat", userId, seatIndex, null);// 2. Place the user in the seat after the invitation is accepted@Overridepublic void onInviteeAccepted(String id, String invitee) {if(id.equals(inviteId)) {mTRTCVoiceRoom.pickSeat(index, null);}}// Listener// 1. The listener receives the invitation@Overridepublic void onReceiveNewInvitation(final String id, String inviter, String cmd, final String content) {if (cmd.equals("pickSeat")) {// 2. The listener accepts the invitationmTRTCVoiceRoom.acceptInvitation(id, null);}}
7. Implement text chat through TRTCVoiceRoom#sendRoomTextMsg.
// Sender: Sends a text chat messagemTRTCVoiceRoom.sendRoomTextMsg("Hello World!", null);// Receiver: Listens for text chat messagesmTRTCVoiceRoom.setDelegate(new TRTCVoiceRoomDelegate() {@Overridepublic void onRecvRoomTextMsg(String message, TRTCVoiceRoomDef.UserInfo userInfo) {Log.d(TAG, "Received a message from" + userInfo.userName + ": " + message);}});
8. Implement on-screen commenting through TRTCVoiceRoom#sendRoomCustomMsg.
// A sender can customize CMD to distinguish on-screen comments and likes.// For example, use "CMD_DANMU" to indicate on-screen comments and "CMD_LIKE" to indicate likes.mTRTCVoiceRoom.sendRoomCustomMsg("CMD_DANMU", "Hello world", null);mTRTCVoiceRoom.sendRoomCustomMsg("CMD_LIKE", "", null);// Receiver: Listens for custom messagesmTRTCVoiceRoom.setDelegate(new TRTCVoiceRoomDelegate() {@Overridepublic void onRecvRoomCustomMsg(String cmd, String message, TRTCVoiceRoomDef.UserInfo userInfo) {if ("CMD_DANMU".equals(cmd)) {// An on-screen comment is receivedLog.d(TAG, "Received an on-screen comment from" + userInfo.userName + ": " + message);} else if ("CMD_LIKE".equals(cmd)) {// A like is receivedLog.d(TAG, userInfo.userName + "liked you.");}}});
Suggestions and Feedback
If you have any suggestions or feedback, please contact colleenyu@tencent.com.